How to send email using .NET and Azure Communication Services
In the previous article we saw how to create authentication credentials for sending emails using SMTP. Now, let's test the credentials created using C# to send an email.
Remember to replace with your domain details and modify the content and recipient details as required.
using System.Net;
using System.Net.Mail;
//Replace with your domain and modify the content, recipient details as required
string smtpAuthUsername = "<Azure Communication Services Resource name>|<Entra Application Id>|<Entra Application Tenant Id>";
string smtpAuthPassword = "<Entra Application Client Secret>";
string sender = "[email protected]";
string recipient = "[email protected]";
string subject = "Welcome to Azure Communication Service Email SMTP";
string body = "This email message is sent from Azure Communication Service Email using SMTP.";
string smtpHostUrl = "smtp.azurecomm.net";
var client = new SmtpClient(smtpHostUrl)
{
Port = 587,
Credentials = new NetworkCredential(smtpAuthUsername, smtpAuthPassword),
EnableSsl = true
};
var message = new MailMessage(sender, recipient, subject, body);
try
{
client.Send(message);
Console.WriteLine("The email was successfully sent using Smtp.");
}
catch (Exception ex)
{
Console.WriteLine($"Smtp failed the the exception: {ex.Message}.");
}
You can visit my GitHub repository to download the source code for this article
For more details, click on the link below to access the official documentation.
How to use SMTP to send an email with Azure Communication Services. - An Azure Communication Services quick start guide.
Learn about how to use SMTP to send emails to Email Communication Services.
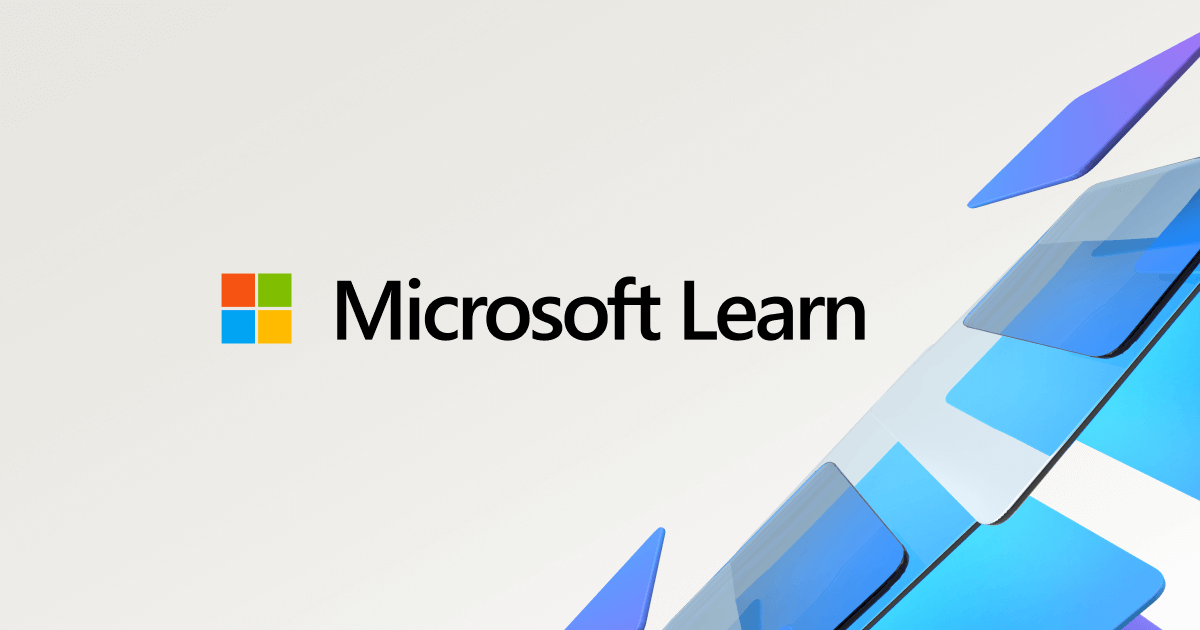
Member discussion