.NET - JSON serialize using snake case and kebab case
.NET 8 has been released and it has built in support for snake case and kebab case naming policies.
Examining the serialized output for each new policy is our next step. To accomplish this, we’re going to use a Person
class defined as follows:
record Person(
int Id,
string FirstName,
string LastName)
This is the code used for testing, in C#, just by changing the PropertyNamingPolicy
parameter for each case.
var person = new Person(Id: 1, FirstName: "Luna", LastName: "Smith");
var options = new JsonSerializerOptions { PropertyNamingPolicy = JsonNamingPolicy.SnakeCaseLower };
var json = JsonSerializer.Serialize(person, options);
SnakeCaseLower (snake_case)
Using JsonNamingPolicy.SnakeCaseLower
as the property naming policy the output will be:
{"id":1,"first_name":"Luna","last_name":"Smith"}
SnakeCaseUpper (SNAKE_CASE)
Using JsonNamingPolicy.SnakeCaseUpper
as the property naming policy the output will be:
{"ID":1,"FIRST_NAME":"Luna","LAST_NAME":"Smith"}
KebabCaseLower (kebab-case)
Using JsonNamingPolicy.KebabCaseLower
as the property naming policy the output will be:
{"id":1,"first-name":"Luna","last-name":"Smith"}
KebabCaseUpper (KEBAB-CASE)
Using JsonNamingPolicy.KebabCaseUpper
as the property naming policy the output will be:
{"ID":1,"FIRST-NAME":"Luna","LAST-NAME":"Smith"}
For more details, click on the link below to access the official documentation
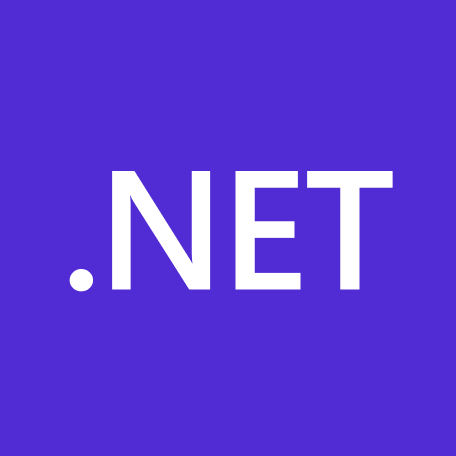
Member discussion