Using the Azure OpenAI SDK with .NET
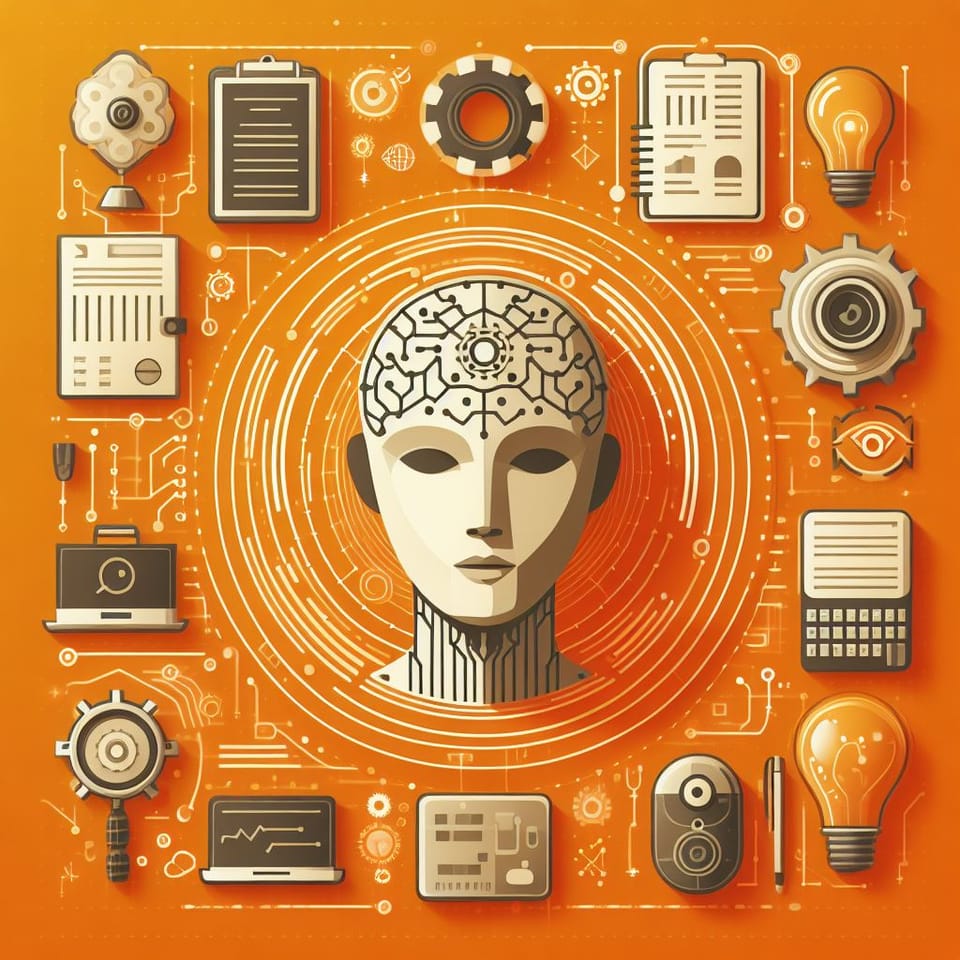
This article will cover how to consume the OpenAI API using the Azure's official .NET library for OpenAI.
Azure.AI.OpenAI 1.0.0-beta.12
Azure’s official .NET library for OpenAI inference that supports Completions, Chat, and Embeddings. Works with Azure OpenAI resources as well as the non-Azure OpenAI endpoint.
For this example, let's ask ChatGPT to act like a real estate agent and create a description about a property.
The first thing to do is go to the OpenAI platform website and get the key to consume the API. You can click here for that.
Once you've got the API key, you can use the C# code below for the API consumption.
using Azure.AI.OpenAI;
// Replace this with a valid api key from Open AI platform
const string nonAzureOpenAIApiKey = "<open-ai-key>";
var openAI = new OpenAIClient(nonAzureOpenAIApiKey, new OpenAIClientOptions());
var completionOptions = new ChatCompletionsOptions
{
// Use the appropriate name of the model (example: gpt-4)
DeploymentName = "gpt-3.5-turbo",
Messages =
{
// The system message represents instructions how the assistant should behave
new ChatRequestSystemMessage("You'll act like you're a real estate agent."),
// This is the prompt
new ChatRequestUserMessage(
@"Write a description of a property with the following characteristics:
The property is an apartment
The sale value is $2,160,000
It has 80m² of living area
It has 100m² of total area
It has 2 parking spaces
It has 4 bedrooms"),
},
MaxTokens = 256,
Temperature = 0.4f,
FrequencyPenalty = 0,
PresencePenalty = 0,
NucleusSamplingFactor = 0
};
var response = await openAI.GetChatCompletionsAsync(completionOptions, CancellationToken.None);
var responseMessageContent = response.Value.Choices[0].Message.Content;
Console.WriteLine(responseMessageContent);
And the response should look like this (or something):
Welcome to this stunning apartment located in the heart of the city, offering a luxurious and contemporary living experience. This exceptional property is now available for sale at the incredible value of $2,160,000.
Spanning across a generous 80m² of living area, this apartment provides ample space for comfortable living. The open floor plan seamlessly connects the living, dining, and kitchen areas, creating a harmonious flow and an ideal space for entertaining guests or enjoying quality time with family.
With a total area of 100m², this apartment offers additional room for relaxation and recreation. The well-designed layout includes four spacious bedrooms, each providing a peaceful retreat for rest and rejuvenation. The abundance of natural light that floods through the large windows creates an inviting and airy atmosphere throughout the entire property.
Convenience is key, and this apartment offers two parking spaces, ensuring that you and your guests will always have a secure place to park. Whether you have multiple vehicles or simply appreciate the convenience of extra parking, this feature is sure to enhance your daily routine.
Situated in a highly sought-after location, this apartment provides easy access to a wide range of amenities, including shopping centers, restaurants, schools, and parks. The vibrant neighborhood offers a vibrant and dynamic lifestyle, with everything you
You can click here to view the source code for this solution.
Member discussion